▣ 자료형(Data Types)
변수의 선언, 할당, 그리고 초기화가 데이터를 어디에 담아서 이용할 것인가와 관련된 내용이었다면, 어떤 유형의 값을 변수에 담을 것인가는 '자료형'과 관련이 있습니다. 또한 자료형은 컴퓨터 과학의 자료 구조(Data Structure)와 밀접한 관련이 있으며, 자바스크립트는 동적 타이핑(Dynamic typing)을 지원하여 어떤 자료형이든 변수에 할당될 수 있습니다.
자료형은 크게 기본형(Primitive)과 참조형(Reference)으로 나뉘며, 단편적으로 설명하자면 스택(Stack) 메모리에 값이 직접 저장되는 것이 기본형이고, 실제 값은 힙(Heap) 메모리에 저장되고 그 주소가 스택 메모리에 저장되는 것을 참조형이라고 합니다. 즉 기본형은 스택 메모리에 접근해서 바로 데이터를 얻고, 참조형은 스택 메모리에 있는 주소 값을 참조해서 데이터에 접근합니다.
그리고 자료형을 확인(Type check)할 때는 typeof 연산자를 사용합니다. 메서드가 아니라는 점에 유의하기 바랍니다.
□ 기본형(Primitive)
/* Primitive Types : string, number, boolean, udefined, null, ... */
// 1. string
const fullName = 'John Doe';
console.log(typeof fullName);
// 2. number
const age = 30;
console.log(typeof age);
// 3. boolean
const hasKids = true;
console.log(typeof hasKids);
// 4. undefined
let job;
console.log(typeof job);
// 5. null
const car = null;
console.log(typeof null); // object
console.log(car === null);
// 6. bigint
const big = BigInt(9007199254740991);
console.log(typeof big);
// 7. symbol
const sym = Symbol('Sym');
console.log(typeof sym);
기본형은 다시 7가지 스트링(String), 넘버(Number), 불리언(Boolean), 언디파인드(undefined), 널(null), 빅인트(BigInt), 심볼(Symbol) 로 나누어집니다. 빅인트와 심볼은 비교적 최근에 추가되었고 주로 보게 될 유형은 바로 스트링, 넘버, 불리언, 언디파인드, 널 5가지입니다.
위의 코드는 각각의 자료형을 간단하게 초기화하고 typeof 연산자를 이용해 각 유형을 확인해 본 것입니다.
※ 스트링, 넘버, 불리언, 언디파인드, 널, 빅인트, 심볼
지금 배우고 있는 부분에 한정하여 단어의 일반적인 의미보다는 자료형의 명칭으로써 음차하도록 하겠습니다.
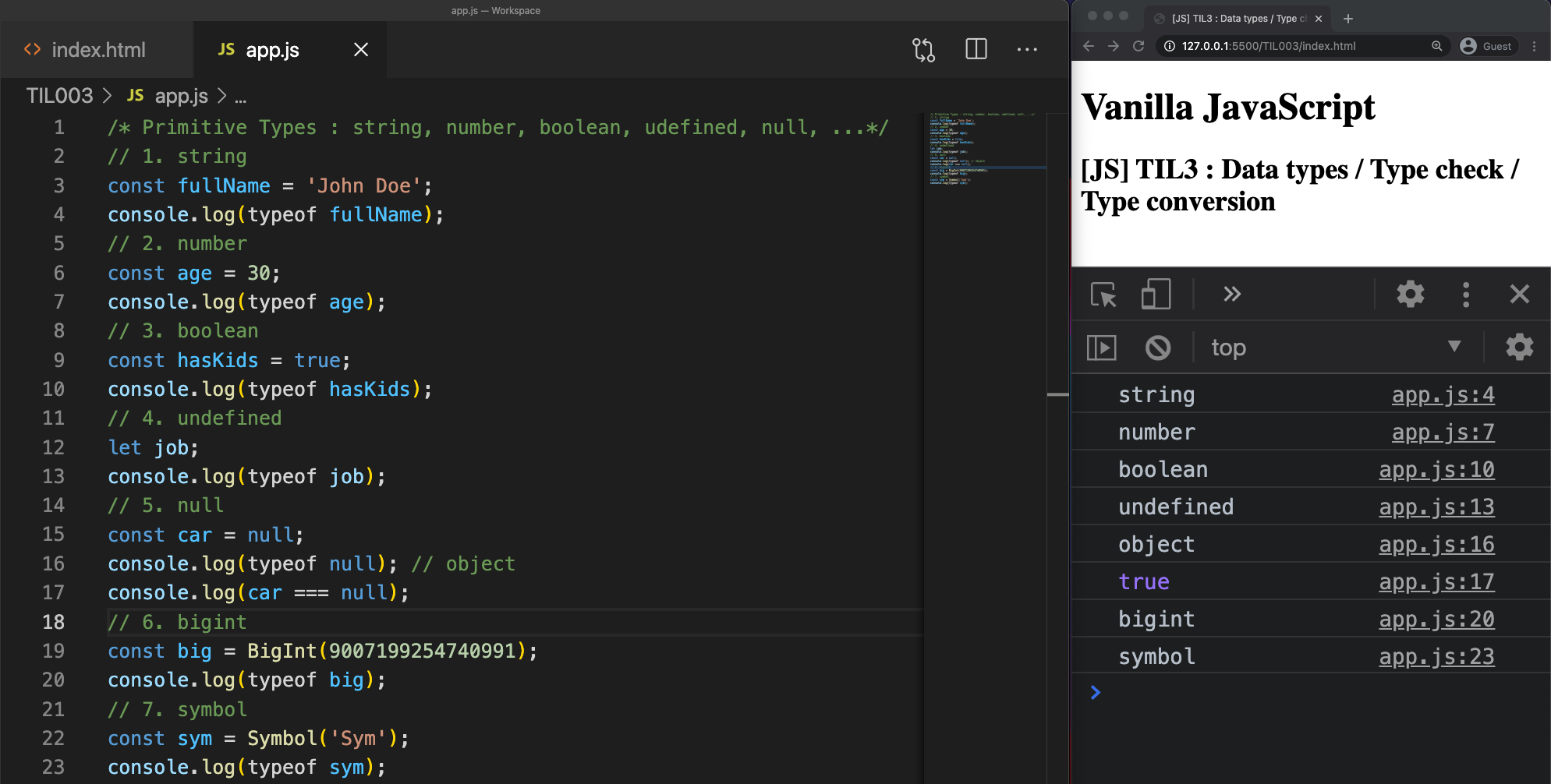
위의 코드 실행 결과를 보면 흥미로운 사실 하나가 있습니다. 20번 라인에서 널 자료형이 'null' 로 출력되지 않고 'object' 로 출력되는 것입니다. 이는 자바스크립트가 만들어질 당시에 typeof 연산자를 정의하면서 발생된 일종의 버그로써, 수정이 요구되었으나 이미 많은 많은 곳에서 프로그래밍에 사용되어 섣불리 손을 댈 수 없기 때문에 그대로 두고 있습니다.
따라서 자료형이 null 인지 확인하기 위해서는 아래와 같이 '===' 기호를 이용하여 직접적인 확인을 해야 합니다.
const test = null;
if (test === null) {
// do this
}
□ 참조형(Reference)
/* Reference Types : object, function */
// 8. object
// ex) Object literal
const address = {
city: 'Seoul',
nation: 'Korea',
};
console.log(typeof address);
// ex) Array
const hobbies = ['movies', 'music'];
console.log(typeof hobbies);
console.log(Array.isArray(hobbies));
// ex) Date
const today = new Date();
console.log(typeof today);
// anything else ...
// 9. function
const hello = function () {
console.log("hello");
};
console.log(typeof hello);
참조형은 다시 2가지 오브젝트(Object), 펑션(Function) 으로 나누어집니다(※ 자료형 명칭으로 한정하여 음차). 오브젝트는 다시 객체(object literal; 자료형 명칭으로써 object가 아님) , 배열, 날짜 등 수많은 유형들이 있으며, 펑션은 연산자 'function'으로 정의된 자료형입니다.
위의 코드와 아래의 실행결과를 보면 기본형과 마찬가지로 typeof 연산자를 이용하여 자료형을 확인할 수 있으며, 특히 배열을 객체와 구분하기 위해서 Array.isArray() 메서드를 사용할 수 있습니다.
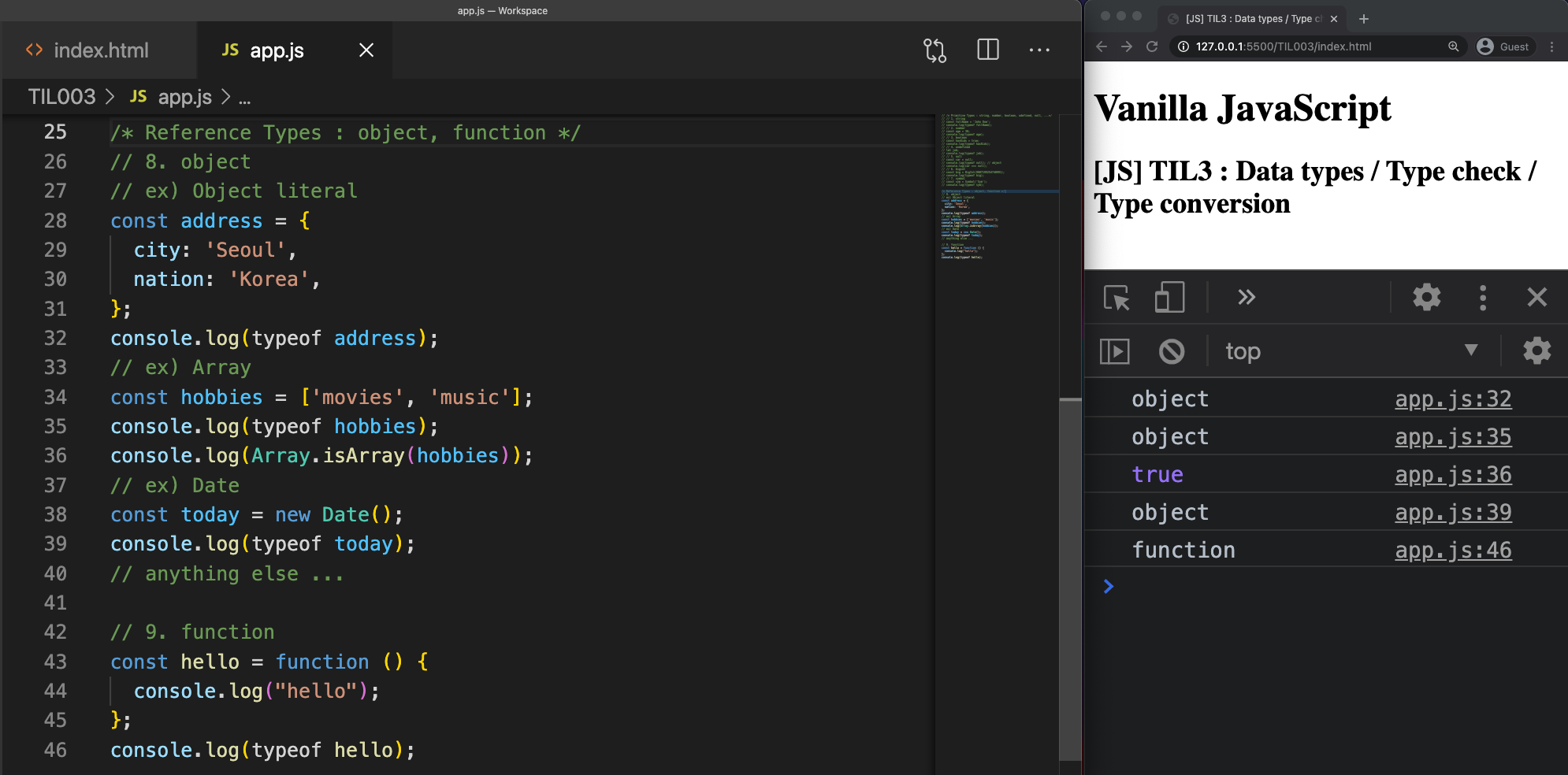
▣ 자료형 변환(Type Conversion)
필요에 따라서는 자료형을 바꾸어 연산을 해야 하는 경우도 있는데, 주로 여러 자료형을 string 또는 number 유형으로 바꾸는 작업을 많이 합니다.
□ 자료형 변환 예시1
let result;
/* Number to string */
result = String(3);
console.log(typeof result);
console.log(result);
console.log(result.length);
/* Bool to string */
result = String(true);
console.log(typeof result);
console.log(result);
console.log(result.length);
/* Date to string */
result = String(new Date());
console.log(typeof result);
console.log(result);
console.log(result.length);
/* Array to string */
result = String([1, 2, 3]);
console.log(typeof result);
console.log(result);
console.log(result.length);
/* toString() : method */
result = (321).toString();
console.log(typeof result);
console.log(result);
console.log(result.length);
스트링 유형이 아닌 자료형을 스트링 유형의 값으로 바꾸는 과정에는 주로 스트링 String() - 자료형 변환함수(객체 생성함수; 일반 함수와 구분하기 위해 대문자를 사용)를 이용하며, 이외에 toString() 메서드도 동일한 역할을 합니다.
아래의 실행 결과에서 볼 수 있듯이 모든 자료형은 string으로 변환되었음을 확인할 수 있습니다. 다만 66번 라인의 배열을 스트링으로 바꾸는 과정에서 양쪽의 기호와 요소들 사이의 공백이 제외되는 것에 유의하기 바랍니다.
※ new String() : 객체 생성자(Constructor)
class 개념이 도입된 이후 new 연산자를 이용하여 객체를 생성할 수 있습니다. 다만 String()과 new String()의 차이점은 각각 기본형(String primitive; 값 자체)과 참조형(String instance object; 인스턴스 객체)을 생성한다는 점입니다.
아래의 코드를 보면, 객체 변환(생성) 함수와 객체 생성자를 이용하여 변환 또는 생성한 데이터의 자료형은 분명히 string과 object로 다름을 확인할 수 있습니다. 반면 나타내는 값은 둘 다 문자열이라서 + 기호나 concat() 메서드를 이용하여 결합이 가능합니다.
/* String Generator Function : 객체 생성함수 */
const a = String(1);
/* String Constructor : 객체 생성자 */
const b = new String(1);
console.log(a === b); // false
console.log(typeof a); // string
console.log(typeof b); // object
console.log(a == b); // true
console.log(a + b); // 11
console.log(a.concat(b); // 11
□ 자료형 변환 예시2
// 위의 코드에 계속 이어서
/* String to number */
result = Number('5');
console.log(typeof result);
console.log(result);
console.log(result.toFixed(2));
result = Number('hello');
console.log(typeof result); // number
console.log(result); // NaN(not a number)
console.log(result.toFixed(2)); // NaN(not a number)
/* Boolean to number */
result = Number(true);
console.log(typeof result);
console.log(result);
console.log(result.toFixed(2));
result = Number(false);
console.log(typeof result);
console.log(result);
console.log(result.toFixed(2));
/* Null to number */
result = Number(null);
console.log(typeof result);
console.log(result);
console.log(result.toFixed(2));
/* Array to number */
result = Number([1, 2, 3]);
console.log(typeof result); // number
console.log(result); // NaN
console.log(result.toFixed(2)); // NaN
/* parseInt() method */
result = parseInt("12.555");
console.log(typeof result);
console.log(result);
console.log(result.toFixed(2));
/* parseFloat() method */
result = parseFloat("12.555");
console.log(typeof result);
console.log(result);
console.log(result.toFixed(2));
넘버 유형이 아닌 자료형을 넘버 유형의 값으로 바꾸는 과정에는 주로 Number() - 자료형 변환함수(객체 생성함수; 일반 함수와 구분하기 위해 대문자를 사용)를 이용하며, 특히 값이 정수나 실수일 경우에는 parseInt(), parseFloat() 메서드를 사용하면 훨씬 다양한 조작이 가능합니다.
아래의 실행 결과에서 볼 수 있듯이 모든 자료형은 number로 변환되었음을 확인할 수 있습니다. 다만 불리언 유형에서 true 값은 1로 변환되고, false 값은 0으로 변환되며, null 유형 또한 그 값이 0으로 변환됨에 유의하기 바랍니다.
그리고 변환 대상이 79번 라인과 같은 숫자 형태의 문자열이 아니라 84번 라인처럼 문자만으로 구성된 값이거나 106번 라인처럼 배열인 경우에는 자료형은 number이지만 그 값이 '숫자가 아님(NaN; Not A Number)'에 주의해야 합니다.
참고로 toFixed()는 number 자료형일 경우에 소수 자리를 나타낼 수 있게 해주는 메서드입니다.
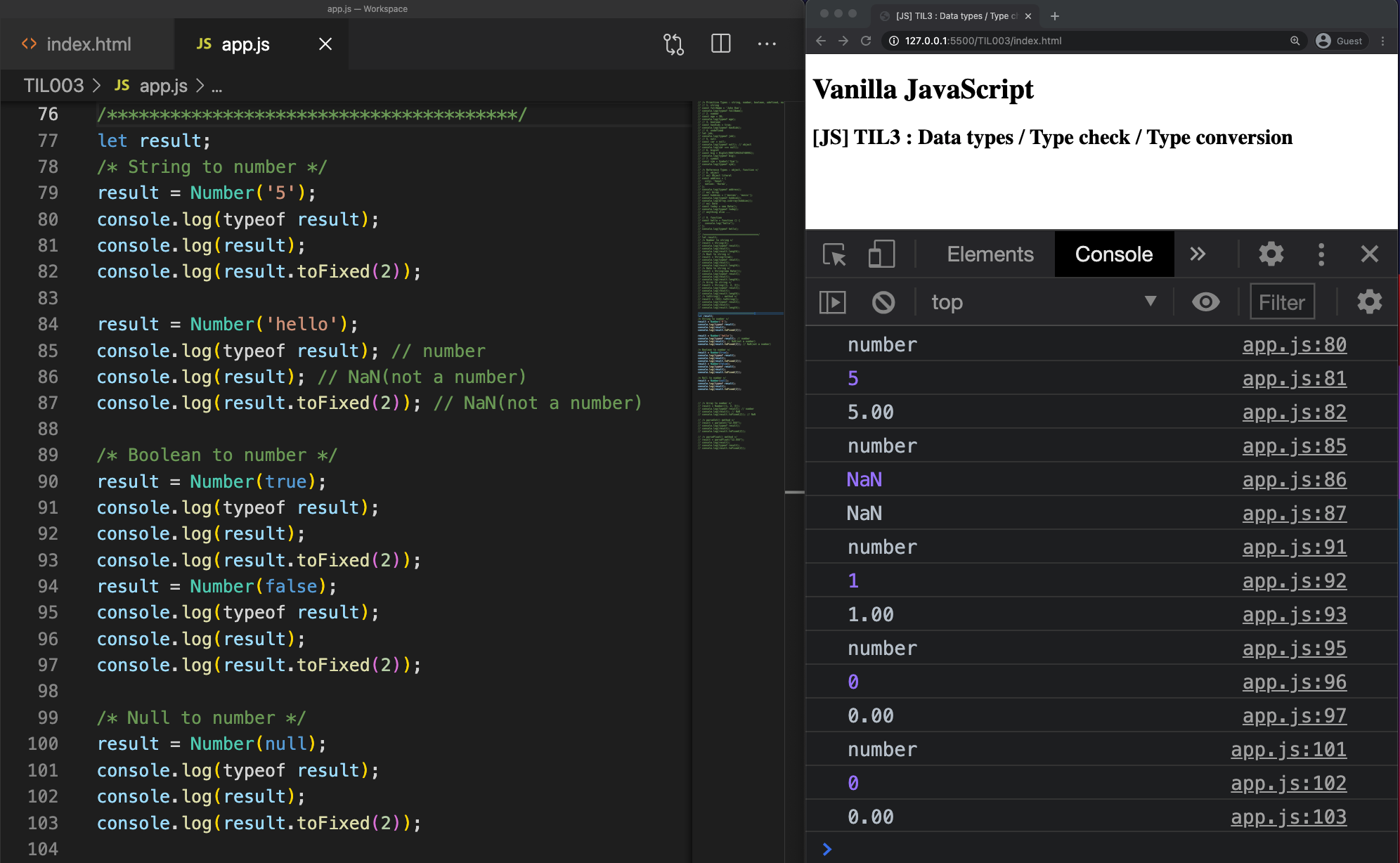
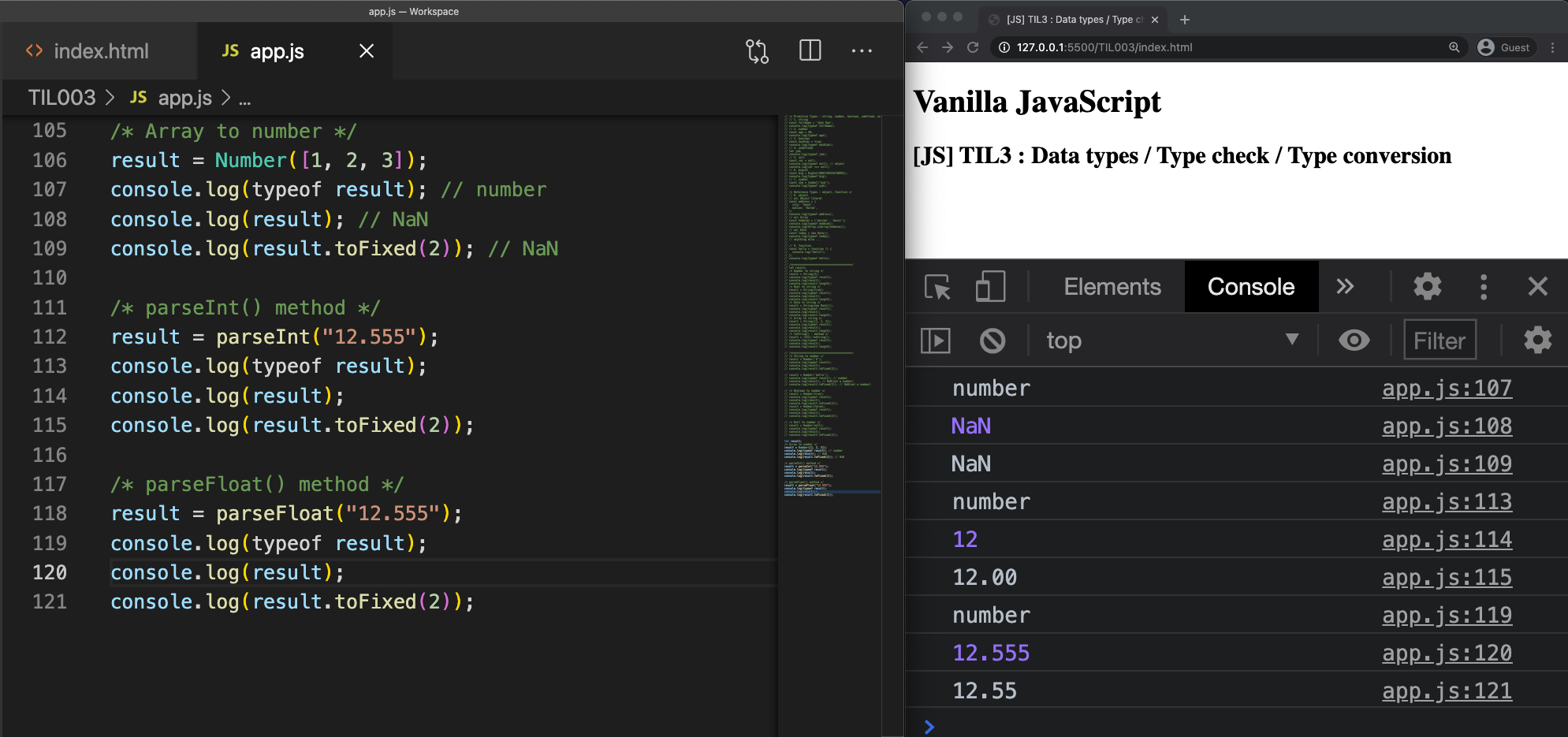
▣ 스트링(String)과 넘버(Number) - 자료형과 값의 구별
/* Number & Number Addition */
let val1 = 5;
let val2 = 6;
let sum = val1 + val2;
console.log(typeof sum);
console.log(sum);
/* Number & String, String & Number Addition */
val1 = 5;
val2 = '6';
sum = val1 + val2;
console.log(typeof sum);
console.log(sum);
val1 = '5';
val2 = 6;
sum = val1 + val2;
console.log(typeof sum);
console.log(sum);
val1 = 'hi';
val2 = 7;
sum = val1 + val2;
console.log(typeof sum);
console.log(sum);
자바스크립트의 자료형에서 짚고 넘어가야 할 나머지 한 가지가 있습니다. 위의 코드를 실행하여 아래의 결과에서 보면, 숫자와 문자열 또는 문자열과 숫자의 덧셈 연산이 가능하고 그 결과는 문자열과 문자열의 결합된 형태와 같습니다. 즉 어느 한쪽이라도 자료형이 string이면 덧셈 연산의 결과는 둘 다 string인 경우와 동일합니다.
이는 '값'과 '자료형'이 엄격히 구분되지 않아 자칫 혼동스러운 상황이 발생될 수 있음을 의미합니다. 물론 이런 특징을 의도적으로 이용하여 코딩을 할 수도 있으나 권장하지는 않습니다.
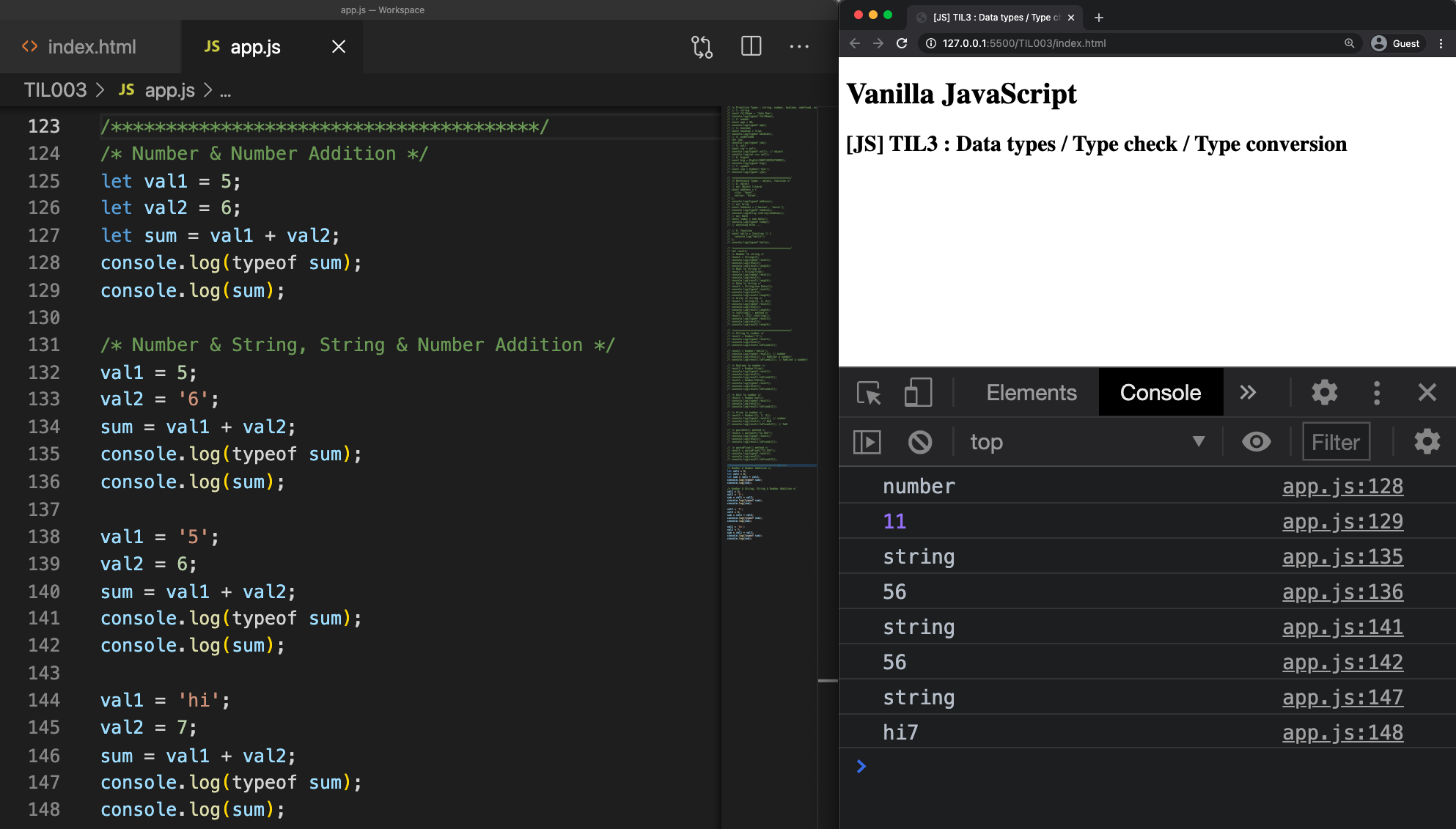
'자바스크립트 > 바닐라 JS' 카테고리의 다른 글
[자바스크립트] TIL5 : 배열 메서드를 이용한 배열의 요소 변경 / 템플릿 문자열의 이용 방법 (0) | 2021.04.11 |
---|---|
[자바스크립트] TIL4 : 스트링 메서드를 이용한 문자열 처리 / 매스 객체를 이용한 수학적 연산 (0) | 2021.04.11 |
[자바스크립트] TIL2 : 변수 선언과 할당 및 초기화 방법 / 변수 영역(스코프)의 정의 (0) | 2021.04.09 |
[자바스크립트] TIL1 : 크롬 웹 브라우저의 자바스크립트 콘솔 이용 방법 (0) | 2021.04.07 |
[자바스크립트] 비주얼 스튜디오 코드와 크롬 웹 브라우저를 이용한 JavaScript, HTML, CSS 코딩 준비 (0) | 2021.04.01 |
댓글